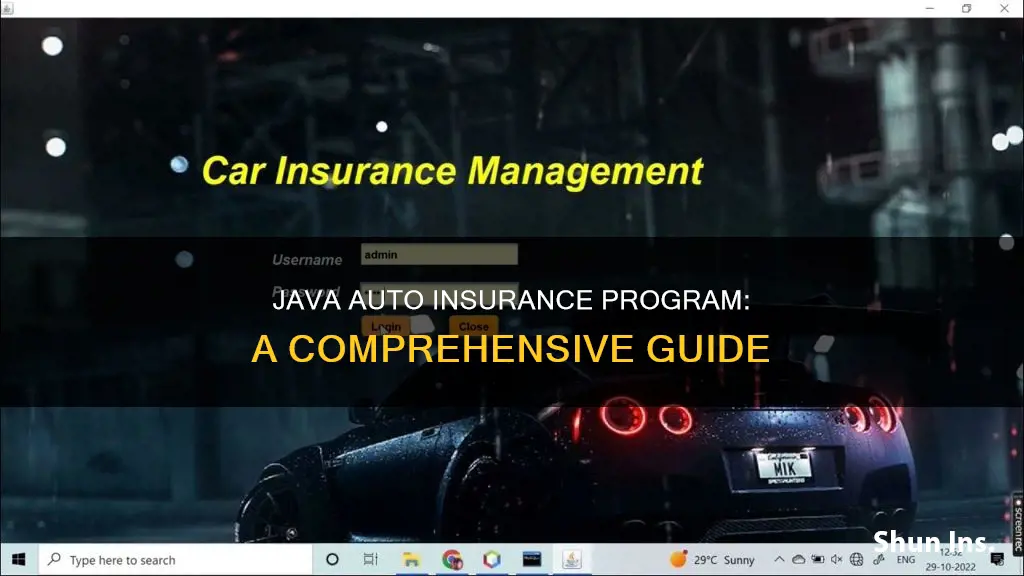
Running an auto insurance program in Java involves creating a console application with an abstract Insurance class and various subclasses. The program should prompt the user for input, such as the type of insurance, and create the appropriate object. The application should also be able to provide information on insurance status, calculate fines for uninsured vehicles, and allow for updating and managing insurance records. The program should utilise variables, methods, and constructors to process and display data effectively.
What You'll Learn
Creating an abstract Insurance class
To create an abstract Insurance class in Java, you can follow these steps and guidelines:
Abstract Class Declaration:
Start by declaring the abstract class using the "abstract" keyword. This indicates that the class cannot be instantiated on its own and needs to be subclassed to use its properties. Here's how you can declare the abstract Insurance class:
Java
Public abstract class Insurance {
// Class properties and methods
}
Class Properties:
Define the properties or attributes of the Insurance class. In this case, you'll need properties such as "policyNumber" and "monthlyPremium." You can also include other relevant properties like the type of insurance, cost, or any other details specific to the insurance program.
Java
Public abstract class Insurance {
Private int policyNumber;
Private double monthlyPremium;
// Other properties
}
Constructors:
Abstract classes can have constructors. Define a constructor for the Insurance class that initializes the properties mentioned above. You can also include parameters in the constructor to set the values of the properties during object creation.
Java
Public abstract class Insurance {
Private int policyNumber;
Private double monthlyPremium;
Public Insurance(int policyNumber, double monthlyPremium) {
This.policyNumber = policyNumber;
This.monthlyPremium = monthlyPremium;
}
}
Getter and Setter Methods:
Create getter and setter methods for the properties of the Insurance class. These methods allow you to access and modify the values of the properties. Include at least one abstract setter method as per the instructions.
Java
Public abstract class Insurance {
// ...
Public int getPolicyNumber() {
Return policyNumber;
}
Public double getMonthlyPremium() {
Return monthlyPremium;
}
Public void setPolicyNumber(int policyNumber) {
This.policyNumber = policyNumber;
}
Public abstract void setMonthlyPremium(double monthlyPremium);
}
Additional Methods:
You can also include other methods in the abstract Insurance class. For example, you might want to include a method to calculate the annual premium based on the monthly premium.
Java
Public abstract class Insurance {
// ...
Public double getAnnualPremium() {
Return monthlyPremium * 12;
}
}
Subclasses:
As mentioned in the instructions, you will need to create subclasses for different types of insurance, such as "Health" and "Life." These subclasses will extend the abstract Insurance class and provide specific implementations for the abstract methods.
Java
Public class Health extends Insurance {
// Subclass implementation
}
Public class Life extends Insurance {
// Subclass implementation
}
Remember to implement the setCost() and display() methods in the subclasses as per the instructions.
By following these steps and guidelines, you can create a well-structured abstract Insurance class for your auto insurance program in Java.
Affordable Auto Insurance: How to Find It
You may want to see also
Adding the Vehicle Identification Number (VIN) property
Understanding the VIN Structure
Before adding the VIN property, it's essential to understand its structure. The VIN is typically composed of 17 characters, including numbers, letters, and dashes. Each section of the VIN provides specific information about the vehicle, such as the world manufacturer identifier (WMI), vehicle descriptor section, check digit, and vehicle identifier section. The VIN can reveal details like the country of origin, manufacturer, model year, vehicle type, and more.
Creating the VIN Property
In your Java code, you need to declare the VIN property within the AutoInsurance class. Here's an example of how you can define the VIN property:
Java
Private String VIN;
Initializing the VIN Property
Once you've declared the VIN property, you need to initialize it in the constructor of the AutoInsurance class. You can pass the VIN as a parameter to the constructor and assign it to the VIN property. Here's an example:
Java
Public AutoInsurance(int policyNumber, double monthlyPremium, double annualPremium, String vin) {
Super(policyNumber, monthlyPremium);
VIN = vin;
AnnualPremium = annualPremium;
}
Implementing Set and Get Methods for VIN
It's essential to provide set and get methods for the VIN property to allow modification and retrieval of the VIN value. Here's how you can define these methods:
Java
Public void setVIN(String vin) {
VIN = vin;
}
Public String getVIN() {
Return VIN;
}
Validating the VIN Format
To ensure the VIN entered by the user is valid, you can implement a validation method that checks the format and length of the VIN. Here's an example:
Java
Public static boolean isValidVIN(String vin) {
// Remove dashes and convert to uppercase
Vin = vin.replaceAll("-", "").toUpperCase();
If (vin.length() != 17) {
Return false; // Invalid length
}
// Check for allowed characters and calculate check digit
// ...
Return true; // Valid VIN format
}
Displaying the VIN in the Output
When displaying the insurance policy details, you should include the VIN information. Update the print() method in the AutoInsurance class to include the VIN:
Java
Public void print() {
DecimalFormat dollar = new DecimalFormat("#,###.00");
System.out.println("Auto Insurance Policy:");
System.out.println("Policy Number: " + getPolicyNumber());
System.out.println("Monthly Premium: $" + dollar.format(getMonthlyPremium()));
System.out.println("Annual Premium: $" + dollar.format(getAnnualPremium()));
System.out.println("VIN: " + getVIN());
}
By following these steps, you can effectively add the Vehicle Identification Number (VIN) property to your auto insurance program in Java. This allows users to input their vehicle's VIN and retrieve relevant insurance information while also ensuring the VIN adheres to the standard format.
Transferring Vehicle Ownership: IAA's Guide to Certificate Title Transfers
You may want to see also
Implementing the print method
To implement the print method in Java for an auto insurance program, you can follow these steps and considerations:
Firstly, you need to understand the purpose of the print method and how it fits into the overall structure of the program. In the provided code, the print method is used to display the details of an auto insurance policy, including the policy number, monthly premium, annual premium, and Vehicle Identification Number (VIN). This method is called after the user selects the type of insurance they require.
The print method should be included in the AutoInsurance class, which extends the abstract Insurance class. This class should have the necessary attributes and methods to store and retrieve information about the auto insurance policy. The print method will utilise these methods to access the required data.
When implementing the print method, you can use the System.out.print() or System.out.println() methods to display the output. The difference between these two methods is that print() keeps the cursor on the same line after printing, while println() moves the cursor to the next line. Here is an example of how you can structure the print method:
Java
Public void print() {
System.out.println("Auto Insurance Policy Details:");
System.out.println("Policy Number: " + getPolicyNumber());
System.out.println("Monthly Premium: " + getMonthlyPremium());
System.out.println("Annual Premium: " + getAnnualPremium());
System.out.println("VIN: " + getVIN());
}
In the above example, the print method uses System.out.println() to display each piece of information on a new line, making the output more readable. The getPolicyNumber(), getMonthlyPremium(), getAnnualPremium(), and getVIN() methods are called to retrieve the respective values for the current auto insurance policy.
You can also format the output using techniques like DecimalFormat to ensure that monetary values are displayed correctly. For example:
Java
DecimalFormat dollar = new DecimalFormat("#,###.00");
System.out.println("Monthly Premium: " + dollar.format(getMonthlyPremium()));
This code snippet formats the monthly premium as a currency value with two decimal places.
Additionally, you may need to consider error handling within the print method. For instance, if the VIN is not available or the premium values are not set, you might want to display a message indicating that certain details are unavailable. You can use conditional statements (if-else) to check for these scenarios and adjust the output accordingly.
By following these guidelines and referring to the provided code, you can effectively implement the print method for the auto insurance program in Java.
Auto Insurance and Attorney Fees: What's Covered?
You may want to see also
Developing a login page
- Create a new project: In your IDE, go to File > New Project, choose the Java type, and enter a project name. Uncheck the "Main class" option.
- Set up the user interface: Drag and drop labels, text fields, and buttons to create the login form. You can use a Null Layout or a Grid Layout for this.
- Add functionality: Implement ActionListener or ActionPerformed methods to handle user input. Compare the entered username and password with predefined values or those stored in a file or database.
- Display messages: Depending on whether the login was successful or not, display appropriate messages to the user.
Java
Import java.awt.*;
Import java.awt.event.*;
Import javax.swing.*;
Public class LoginDemo extends JFrame implements ActionListener {
JPanel panel;
JLabel userLabel, passwordLabel, messageLabel;
JTextField userNameText;
JPasswordField passwordText;
JButton submitButton, cancelButton;
Public LoginDemo() {
UserLabel = new JLabel("User Name:");
UserNameText = new JTextField();
PasswordLabel = new JLabel("Password:");
PasswordText = new JPasswordField();
SubmitButton = new JButton("Submit");
Panel = new JPanel(new GridLayout(2, 2));
Panel.add(userLabel);
Panel.add(userNameText);
Panel.add(passwordLabel);
Panel.add(passwordText);
Panel.add(submitButton);
SubmitButton.addActionListener(this);
SetDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Add(panel);
SetTitle("Login Page");
SetSize(400, 300);
SetVisible(true);
}
Public static void main(String[] args) {
New LoginDemo();
}
@Override
Public void actionPerformed(ActionEvent e) {
String userName = userNameText.getText();
String password = passwordText.getText();
If (isValidUser(userName, password)) {
MessageLabel.setText("Login successful!");
} else {
MessageLabel.setText("Invalid username or password.");
}
}
Private boolean isValidUser(String userName, String password) {
// Check the username and password here
// Return true if valid, false otherwise
Return false; // Replace with your validation logic
}
}
This code sets up a basic login form with labels, text fields, and buttons. The `actionPerformed` method is called when the submit button is clicked, and it checks if the entered username and password are valid. You would need to implement the `isValidUser` method to define how you want to validate the user's credentials.
Remember to handle exceptions and ensure that sensitive data, like passwords, is securely stored and transmitted.
Auto Insurance Approval: How Long Does It Take?
You may want to see also
Displaying insurance types and costs
To display insurance types and costs in a Java auto insurance program, you can follow these steps and guidelines:
Firstly, create an abstract superclass named "Insurance" that will serve as the foundation for different types of insurance policies. This superclass should include instance variables such as "insuranceType" and "monthlyCost" to describe the type of insurance and its monthly cost, respectively. Implement getter methods for these variables to retrieve their values. Additionally, declare abstract methods within the Insurance class, such as "setCost()" and "display()". These methods will be implemented by the subclasses.
Next, create subclasses for different types of insurance, such as "Health" and "Life". These subclasses will extend the Insurance class and provide specific implementations for the setCost() and display() methods. For example, in the Life subclass, you might set the monthly cost to a fixed value of $36, while in the Health subclass, you set it to $196. The display() method can be used to show relevant information to the user, such as the insurance type, policy details, and costs.
When creating the user interface, prompt the user to select the type of insurance they are interested in. Based on their input, create an instance of the corresponding insurance subclass (Health or Life). This allows the program to associate the selected insurance type with its respective cost and display the information to the user.
To display the insurance types and costs, you can use a polymorphic screen manager. This manager will iterate through an array of insurance references, sending setCost() messages to each object and displaying the information on the screen. This way, the user can see the different insurance options along with their associated costs.
Java
Import java.util.Scanner;
Public class InsuranceDisplay {
Public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Select insurance type:");
System.out.println("1. Health");
System.out.println("2. Life");
Int choice = input.nextInt();
If (choice == 1) {
HealthInsurance health = new HealthInsurance();
System.out.println("Health Insurance:");
System.out.println("Monthly Cost: $" + health.getMonthlyCost());
} else if (choice == 2) {
LifeInsurance life = new LifeInsurance();
System.out.println("Life Insurance:");
System.out.println("Monthly Cost: $" + life.getMonthlyCost());
} else {
System.out.println("Invalid choice. Please select a valid insurance type.");
}
}
}
Abstract class Insurance {
Protected String insuranceType;
Protected double monthlyCost;
Public Insurance(String type) {
This.insuranceType = type;
}
Public abstract void setCost();
Public abstract double getMonthlyCost();
}
Class HealthInsurance extends Insurance {
Public HealthInsurance() {
Super("Health");
SetCost();
}
@Override
Public void setCost() {
MonthlyCost = 196.0;
}
}
Class LifeInsurance extends Insurance {
Public LifeInsurance() {
Super("Life");
SetCost();
}
@Override
Public void setCost() {
MonthlyCost = 36.0;
}
}
In this example, the program prompts the user to select an insurance type and then displays the corresponding insurance information, including the monthly cost. The "Insurance" class is an abstract superclass, and "HealthInsurance" and "LifeInsurance" are its subclasses. Each subclass overrides the "setCost()" method to set the monthly cost specific to that type of insurance.
Does Your Auto Insurance Cover You in Mexico?
You may want to see also